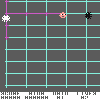
First the simple static array. Static because it can only contain a fixed amount of items; Simple because we only put strings in it referred by the indexnumber (or subscript) of the array:
' Simple static array
Dim weekdays(6)
weekdays(0) = "Mon"
weekdays(1) = "Tue"
weekdays(2) = "Wed"
weekdays(3) = "Thu"
weekdays(4) = "Fri"
weekdays(5) = "Sat"
weekdays(6) = "Sun"
When you don't have plans to use the subscript, you can also create the array directly. And yes, it works the same with index numbers as with the weekdays array, but it is good programming practice to use the former method if you want to call the array items by subscript number.
' Simple assigned array
Dim workdays
workdays = array("Mon", "Wed", "Thu", "Fri")
As you can see, the variable does not have to be declared as an array, so theoretically every variable can be set as an array just as every variable can be set as an object. Although, with arrays you don't need the set statement.
Sometimes it is more convenient to dynamically increase and decrease the array size. Then you have to create a dynamic array. This is an array declared the same way as in the simple static array, but without the number of elements, just empty parenthesis: dim myArray()
To set the amount of array items, you have to use ReDim like ReDim myArray(n) where n is the amount of items you want to use +1 (The first subscript is always 0). Other then in a static array declaration (like Dim myArray(5)), the number of subscripts in a ReDim statement can be a variable or constant.
Whenever you use ReDim, the array is reïnitialized, except when you use the Preserve command, indicating you want to reuse the already set values:
' Simple dynamic assigment of an array
Dim myPets()
ReDim myPets(2)
myPets(0) = "Dog"
myPets(1) = "Cat"
myPets(2) = "Hippopotamus"
ReDim Preserve myPets(3)
myPets(3) = "Rabbit"
msgbox "My pets: " & join(myPets, ", ")
The join(array[, separation character(s)]) function lets you easily merge an array to a string.
Another trick is to use join to make an html table easily:
newTableRow = "" & join(arrElements, "") & ""
Multidimensional arrays
A multidimensional array is used to store data or objects in a matrix. You can create virtually create as much dimensions if you want (not really unlimited of course, but keep in mind this good rule with programming: If you have to ask what the limit is for some kind of instance, probably there is something wrong with your design)
' Multidimensional static array, the next code is pure and alone for demonstration purposes
' it is not optimised, maybe even not correct and there are better ways to achieve this
Dim bcCalendar(2100, 12, 31)
Dim dayCounter, maxDay, cYear, cMonth, cDay
dayCounter = 6
For cYear = 0 to 2100
For cMonth = 1 to 12
Select Case cMonth
Case 1,3,5,7,8,10,12 maxDay = 31
Case 4,6,9,11 maxDay = 30
Case 2 maxDay = 28 + abs((cYear mod 400 = 0) or ((cYear mod 4 = 0) and not (cYear mod 100 = 0)))
End Select
For cDay = 1 to maxDay
bcCalendar(cYear, cMonth, cDay) = weekday((dayCounter mod 7)+1)
dayCounter = dayCounter + 1
Next
Next
Next
msgbox "Charles Darwin was born on a " & WeekdayName(bcCalendar(1809, 2, 12))
Passing arrays
Arrays are passed the same way as variables are:
Default: By Reference
With ByRef in the function declaration: By Reference
With ByVal in the function declaration: By Value
With parenthesis around the argument in the function call: always By Value (ByRef in the function declaration is omitted)
Arrays filled with objects
Arrays do not only have to contain variables, they can also contain objects, which is great to make a collection of QTP gui objects, dictionaries, but also class objects to create child classes under a parent.
Arrays can even contain other arrays. This is useful if you want to create a fully dynamic multidimensional array. (Normally, in a multidimensional array, only the last item is expandable with a redim preserve statement.)
No comments:
Post a Comment